Creating a Simple Arduino Fire Detector
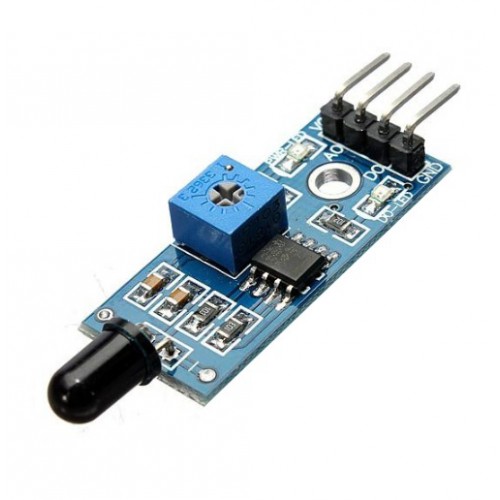
A fire or flame sensor is simply an infrared LED with an amplifier circuit. Flame radiates an infrared signal, which triggers the LED to produce current. This current is converted to voltage for an analog-to-digital converter to read. Let's see how we can build an Arduino fire detector using this sensor.
Flame Sensor Module
For this tutorial, I’ll be using the flame sensor module:
This module has four outputs as shown: VCC, GND, D0, and A0. It also has two LEDs, one is for power indication and one is directly tied to D0. A trimmer is also present which is used to adjust the sensitivity of this flame sensor.
Basically, the D0 pin goes high when a flame intensity threshold is reached. The threshold can be varied by turning the trimmer. The A0 pin produces voltage representing the measured flame intensity.
There are two ways to use the fire detector with Arduino. One is to use the D0 pin and check whenever the pin goes high. A simple sketch such as this can be used:
int sensorPin = 2; // D0 pin to Arduino’s D2 pin void setup() { pinMode(sensorPin, INPUT); Serial.begin(9600); } void loop(){ if(sensorPin == HIGH){ Serial.println("Fire Detected"); delay(1000); } }
If you want to use the A0 pin, just connect it to any analog pin. However, you need to manually check which values provided by the ADC means there’s a fire.
Let’s say you’ve done the test and determined that a value less than 100 indicates there’s a fire. You can now use this sketch:
int sensorPin = A0; // A0 pin to Arduino’s A0 pin void setup() { pinMode(sensorPin, INPUT); Serial.begin(9600); } void loop(){ if (sensorPin < 100){ Serial.println("Fire Detected"); delay(1000); } }
Now you have a simple Arduino fire detector! Also, using this flame sensor with a smoke detector plus buzzers gives you a simple fire alarm system!