Digital Thermometer with Nokia 3310 LCD
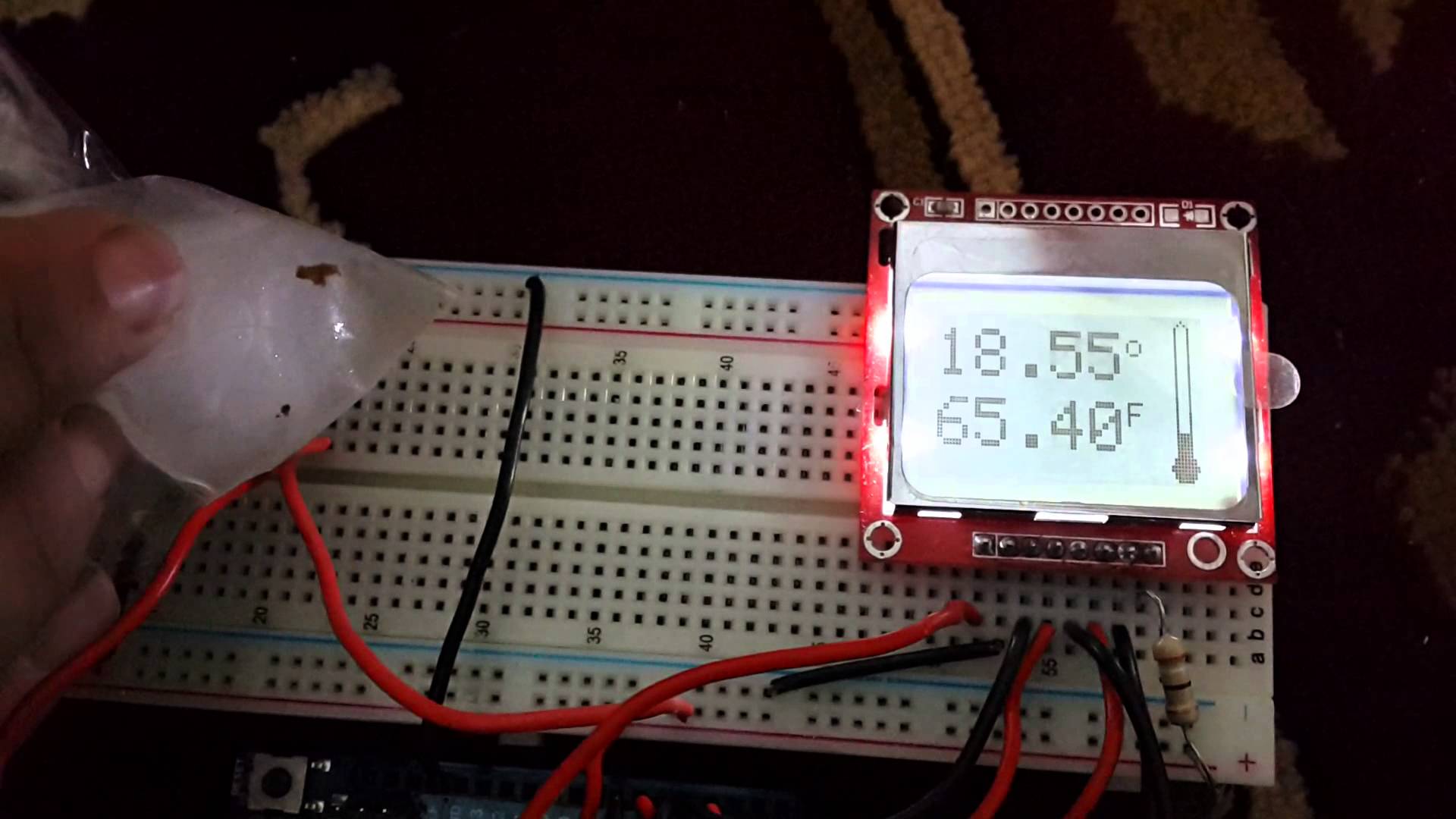
The Nokia 3310 LCD is a cheap option for those who want to add a graphical display to their microcontroller projects. This digital thermometer project uses this LCD to display the temperature from a LM35 temperature sensor.
Introduction
A temperature sensor is a fairly common microcontroller project. The sensing device in this project is a LM35 integrated circuit that gives 10mV per degree celsius and can detect from 0 degree celsius to 100 degree celsius.
The PIC16F877A microcontroller used in this project has 10-bit ADC and a minimum sensible voltage of 4.88 mV. This means the conversion factor for the LM35 should be 10mV/2.88mV or 2.046. This value is used in the code to convert the ADC value to temperature in degree celsius.
The Nokia 3310 LCD uses SPI for interfacing with a microcontroller. I’ve created two libraries for the PIC16F877A to communicate with this LCD and is included in the downloadable design files.
Materials Needed
- PIC16F877A
- Nokia 3310/5110 LCD Breakout Board
- LM35
- 4.7 uF Mylar Capacitor
- SPST Pushbutton
- 4 MHz Crystal
- 2 x 22pF Ceramic Capacitor
Schematic Diagram
This project was tested and simulated using Proteus ISIS. You will need the Nokia 3310 LCD Proteus library to do your own simulations.
Code
/* * File: nokia_thermometer.c * Author: Roland Pelayo * For Digital Thermometer with Nokia LCD Project * * Created on April 4, 2018, 3:55 PM */ #pragma config FOSC = XT // Oscillator Selection bits (XT oscillator) #pragma config WDTE = OFF // Watchdog Timer Enable bit (WDT disabled) #pragma config PWRTE = OFF // Power-up Timer Enable bit (PWRT disabled) #pragma config BOREN = OFF // Brown-out Reset Enable bit (BOR disabled) #pragma config LVP = OFF // Low-Voltage (Single-Supply) In-Circuit Serial Programming Enable bit (RB3 is digital I/O, HV on MCLR must be used for programming) #pragma config CPD = OFF // Data EEPROM Memory Code Protection bit (Data EEPROM code protection off) #pragma config WRT = OFF // Flash Program Memory Write Enable bits (Write protection off; all program memory may be written to by EECON control) #pragma config CP = OFF // Flash Program Memory Code Protection bit (Code protection off) #define _XTAL_FREQ 20000000 #include <xc.h> #include <stdio.h> #include <conio.h> #include <stdlib.h> #include "pcd8544.h" int temp = 0; unsigned int ADC_read(int channel){ ADFM = 1; //results right justified ADCS0 = 0; //conversion speed = 4*Tosc ADCS1 = 0; ADCS2 = 1; ADCON0bits.CHS = channel; ADON = 1; //turn on ADC __delay_ms(1); GO_DONE = 1; while(ADCON0bits.GO_DONE == 1); unsigned int adval = (ADRESH << 8) + ADRESL; // return adval; } void main(void) { lcdBegin(); //initialize LCD lcdClear(); //clear LCD screen lcdCursor(0, 0); //place cursor at x=0,y=0 lcdPrint("Temperature: "); while(1){ int adval = ADC_read(0); //read signal from LM35 char buf[5]; //buffer to hold ADC value itoa(buf, adval/2.046, 10); //convert integer (now in celsius) to string lcdCursor(25, 2); //place cursor at x=25,y=2 lcdPrint(buf); //print temperature value lcdPrint(" C"); __delay_ms(1000); //some delay } return; }
As mentioned, this project requires two libraries, pcd8544.h and SPI.h, to work. They are included in the downloadable files.
The signal from the LM35 is easily converted to readable data using analog to digital conversion. In my code, the reading is done every second. You can adjust the delay for the code according to your needs.